Last winter, Jan Karel and I worked a little on the QueryManager add-in. I was interested in that project because I wanted an easy way to add a parameter to an external data query. After the first round, the method I wrote to add a parameter was just too hokey. It merely added a criterion with a question mark, and didn’t give the user a whole lot of options. Once the question mark was added to the SQL, it was up to the user to define the parameter in the UI. We needed something that did the whole job.
On subsequent rounds, everything was getting complicateder and complicateder. I ended up just parsing the whole SQL statement to try to accomplish what I wanted. The problem was I don’t know SQL well enough to be parsing it for a general purpose utility. From what I read, even people who do know SQL found the task difficult. I like the utility that Jan Karel developed, but I was never (and am not) satisfied with my end of it.
I should just quit thinking about, but it haunts me. My latest foray into SQL Parsing was going to be brilliant. I was going to use DAO automation to create a query in Jet, then read the contents of the MSysQueries table (a hidden table in Access). If you use queries in Access and you want to see how Access stores the details, go to Tools > Options and check the Hidden Objects check box. Then open the MSysQueries table and you can see the various components of a query. I wanted to put this table into a recordset so I could manipulate the pieces and reassemble them, but I ran into access restrictions. I don’t know enough about Access security to even understand the problem, much less the solution.
I was perusing the References dialog in the VBE earlier. I was actually looking for a mozilla dll to see what I could do with it and came upon this:
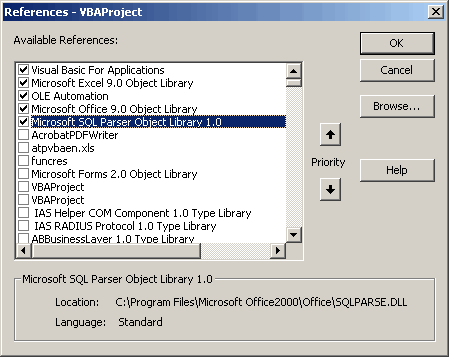
An SQL parsing library? Sweet! I haven’t got it all figured out and from what I’ve done, I wouldn’t necessarily call this robust. It seems to be a step in the right direction, though.
Sub TestParse()
Dim prsApp As MSSQLParser.vbSQLParser
Dim sSql As String
Dim sTemp As String
Dim vaParsed As Variant
Dim i As Long
Const sSPLIT As String = “”
Set prsApp = New MSSQLParser.vbSQLParser
sSql = “SELECT * FROM TblCustomers WHERE TblCustomers.CustId = ‘1234’ “
sSql = sSql & “AND TblCustomers.State = ‘NE'”
sTemp = prsApp.ParseSQLSyntax(sSql, vbSqlServerSyntax)
vaParsed = Split(sTemp, sSPLIT)
For i = LBound(vaParsed) To UBound(vaParsed)
Debug.Print i, vaParsed(i)
Next i
End Sub

I don’t have this thing quite figured out, but here’s what I’ve gathered so far.
- cf1 = SQL keywords like SELECT, FROM, WHERE
- cf5 = punctuations, mathematical operators, and logical operators
- cf6 = string literals
- cf7 = numeric literals
- cf10 = This appears to be a catch all. I thought it was table names and fields, but after misspelling SELECT, it put it as cf10. So now I think this is just anything it doesn’t recognize.
Kind of a strange parsing system, I think. Not really as useful as MSysQueries, but at least it can identify SQL keywords, which is more than I can say for myself. I’m not sure if this will turn into something useful for me, but it has renewed my interest in our QueryManager project. Now I need to figure out how to get Rob involved and take the best of his Query Editor program and ours.